When using notifications in Dynamics AX we get different event types when setting up a new notification rule for different fields. If we try to create a notification rule for a date field, we get event types like “is due:”, “is due in:” and “has changed:”. If we create one for a string we get “has changed:” and “is set to:”.
Let’s say we want to create a new event type for fields containing numbers that checks if the number has doubled. This is how we do it.
We first need to create a new class that extends the EventTypeCUD class. We call our new class EventTypeCUDDoubleOrMore.
Classdeclaration extends EventTypeCUD:
1 2 3 | class EventTypeCUDDoubleOrMore extends EventTypeCUD { } |
We need a description that tells the user what the event type means. This is what the user will see then choosing appropriate event type.
1 2 3 4 | public EventTypeDescription description() { return "has doubled:"; } |
We need to specify what type of fields our new event type will be applied to:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | public boolean isValidEventType(Types _types) {; switch(_types) { case Types::Integer: case Types::Int64: case Types::Real: return true; default: return false; } return false; } |
We also need a condition that will trigger a notification if our event is used:
1 2 3 4 | public boolean isMatchingRule() { return (currentValue >= (originalValue * 2)); } |
And last but not least we need to specify that this event has the trigger FieldChanged.
1 2 3 4 5 6 7 8 9 | public anytype parmTypeTrigger(EventTypeTrigger _typeTrigger = typeTrigger) { ; typeTrigger = _typeTrigger; if (typeTrigger != EventTypeTrigger::FieldChanged) throw error("@SYS96349"); return typeTrigger; } |
More info on how to create a new event type class can be found in Dynamics AX developer help chapter: Implement a New EventType Class.
What they don’t mention in the developer help is how to activate your new class. This is done in the class EventType method eventTypeClassIdsHook(). There are comments in the code that helps, we need to edit this method so it looks like this:
1 2 3 4 5 6 7 | protected static container eventTypeClassIdsHook() { // return connull(); return [classNum(EventTypeCUDDoubleOrMore)]; // return [classNum(myEventTypeClass1), // classNum(myEventTypeClass2), ... ]; } |
Result:
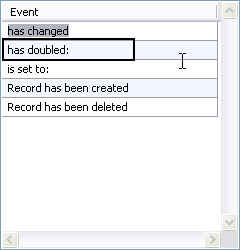
Last tip, you don’t have to extend your class to EventTypeCUD, if you are going to create an event type that is similar an existing one, it might be a good idea to extend that class instead. That way you don’t have to create some of the methods like parmTypeTrigger() and isValidEventType().
Last 5 posts in Development
- No valid document identified from the entity key - April 9th, 2010
- Using Regular Expressions in Dynamics AX - October 2nd, 2009
- Create class and methods in x++ - December 22nd, 2008
- Simple field lookup in form - October 13th, 2008
- Get your Intercompany CustAccount - September 29th, 2008